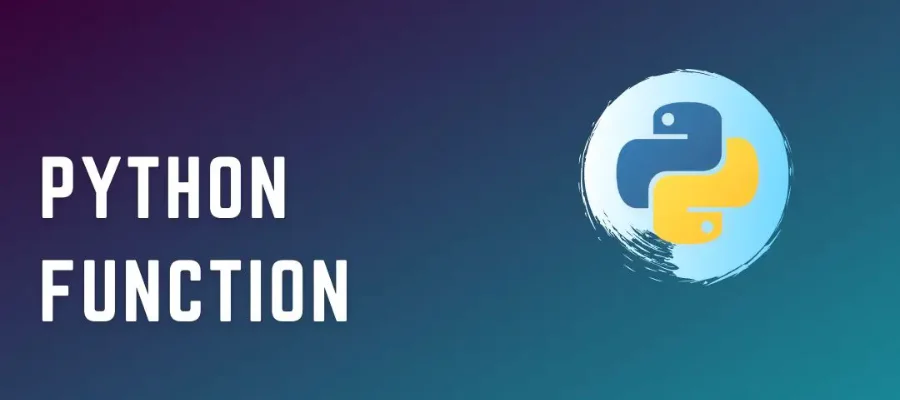
Python Function | Hermagic
In Python, Functions are used to break down larger programs into smaller, more manageable pieces. They also help in code reusability, which means that a block of code can be used multiple times throughout the program without having to rewrite it each time. Python functions can be called at any point in the program, and they can also return values, making them versatile tools for solving complex programming problems. Read on to learn more about the functions in python and lambda function python.
Defining a Python Function
In Python, a function is defined using the “def” keyword followed by the name of the function and a set of parentheses. The parentheses can contain optional parameters that the function uses to perform its task. The function body is indented and can contain any number of statements, including conditional statements, loops, and other functions.
Here’s an example of a simple Python function that prints a message:
def print_message():
print(“Hello, world!”)
In this example, we defined a function called “print_message” that doesn’t take any arguments and simply prints the message “Hello, world!” when called.
Function parameters and arguments
Python functions can also take one or more parameters, which are placeholders for data that the function needs to perform its task. When a function is called, the values that are passed to the parameters are called arguments. Parameters and arguments can be of any data type, including strings, integers, floats, and more.
Here’s an example of a Python function that takes two parameters and returns their sum:
def add_numbers(x, y):
return x + y
In this example, we defined a function called “add_numbers” that takes two parameters, “x” and “y”. The function body returns the sum of these two parameters.
Calling a Python function
To call a Python function, we simply use the function name followed by parentheses. If the function takes arguments, we pass them inside the parentheses.
Here’s an example of calling the “print_message” function we defined earlier:
print_message()
When we call this function, it will print the message “Hello, world!” to the console.
Here’s an example of calling the “add_numbers” function with arguments:
result = add_numbers(3, 5)
print(result)
In this example, we called the “add_numbers” function with the arguments 3 and 5. The function returned their sum, which we stored in a variable called “result”. Our next step was to output the number of “result” to the console.
Returning values
Python functions can also return values using the “return” keyword. When a function returns a value, it can be assigned to a variable or used in any other way that a value of that data type can be used.
Here’s an example of a Python function that returns the square of a number:
def square(x):
return x ** 2
In this example, we defined a function called “square” that takes one parameter, “x”. The function body returns the square of “x”.
We can call this function and assign its return value to a variable like this:
result = square(4)
print(result)
In this example, we called the “square” function with argument 4. The function returned the square of 4 (which is 16), which we stored in a variable called “result”. Following that, we output to the console the number that was stored in the “result” variable.
What is the built-in python function?
In Python, built-in functions are functions that are available in the standard library of the language. These functions are predefined and can be used directly in a program without requiring any additional import statements or library installations. Built-in functions are designed to provide commonly used functionalities that are required in most programs. These functions are optimized for performance, and they are implemented in C or Python itself, making them fast and efficient.
Why are built-in functions important?
Built-in functions are important in Python programming for several reasons.
Save time and effort
Firstly, they save time and effort by providing pre-defined functionality, which can be used directly in a program without any prior declaration or definition.
Optimization
Built-in functions are optimized for performance, which means that they execute faster than user-defined functions. This is because built-in functions are implemented in C or Python itself, making them more efficient.
Easy to understand
Built-in functions provide a standardized way of performing common tasks, which makes code more readable and easier to understand. This is because built-in functions have consistent names and syntax, which makes them easier to recognize and use.
Conclusion
Python functions offer many benefits to programmers. They can be used to break down large programs into smaller, more manageable pieces, making it easier to maintain and update the code. Additionally, functions allow for code reuse, which can save a significant amount of time and effort in the long run. When designing functions, it is important to consider factors such as input parameters, output values, and error handling to ensure that they are robust and reliable. Testing functions thoroughly is also critical to ensure that they work as intended and produce accurate results. For more information, visit Hermagic.
FAQ’s
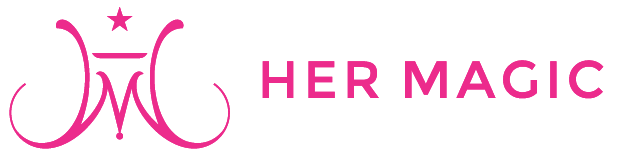